Table of Contents
Introduction
For years, ReactJS has been the industry standard, and because it has a promising future, developers flock to this tool to advance their careers. So, if you are one of them and want to ace the interview, here are the top 25 ReactJS interview questions and answers that interviewers commonly ask, ranging from freshers to senior level.
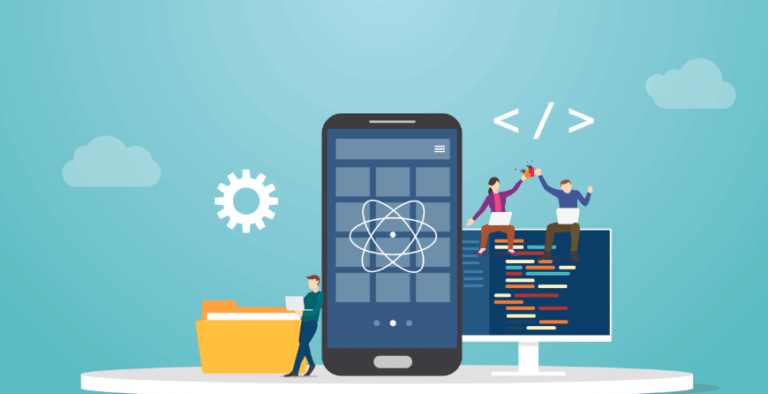
How Do I Prepare For ReactJS Interview?
No matter if you are a fresher or an expert ReactJS developer, everyone asks this question.
However, to give you a brief idea of how to prepare for a ReactJS interview, let’s take a look at the ReactJS interview topics that you should cover.
- 1. DOM and Virtual DOM.
- 2. React LifeCycle Methods.
- 3. JSX.
- 4. Controlled, Uncontrolled Components, and Pure Components.
- 5. Redux.
- 6. HOC.
- 7. State Props Ref
- 8. Hooks.
- 9. Forms.
- 10. Classes.
- 11. ES6.(If you are unaware of ES6)
Although the above topics are crucial to ace the interview, a developer must expect the unexpected and study all the topics and also practice coding problems that may get asked in the interview.
ReactJS Interview Questions And Answers For Freshers
What is React?
Launched in 2011 by Facebook, React is a JavaScript library for building user interfaces for the front end based on UI components. Its component-based approach allows developers to build UI elements with high reusability.
Today, it’s used to design the UI for both mobile and desktop applications.
What are the features of ReactJS?
ReactJS offers some unique features, and some of the most important features are mentioned below.
- Virtual DOM, which is useful for rendering UI at a faster speed.
- ReactJS makes use of a unidirectional data flow model.
- ReactJS has JSX which provides ease to developers to write building blocks.
What is Virtual DOM?
A virtual DOM is a JavaScript object in which the structured representation of a user interface is stored in memory and synced with a real DOM using the ReactDOM library.
It’s a copy of the real DOM and acts as a node tree consisting of attributes, elements, and other properties. It creates the node tree with the help of the ReactJS render function and it gets updated as changes occur in the data model.
What is JSX?
JSX is used in ReactJS to describe what the UI should look like. It is a syntax extension of JavaScript and it allows developers to combine HTML and JavaScript in the same file, which improves page readability and the performance of the application.
Why is ReactJS so Widely Used?
ReactJS is widely used because it provides developers with some advantages while building an application.
- It offers reusable components, which can be used for multiple new projects.
- Faster UI rendering with Virtual DOM.
- React makes testing easy.
- React is easy to scale.
- Provides higher readability to users.
- React is suited for both client and server sides.
Can Browsers Read JSX?
No, browsers can’t read JSX. They only read JavaScript object files, but to make a browser read JSX files, they must be compatible with JavaScript first.
Furthermore, they have to be transformed into JavaScript objects with the help of a JSX transformer like Babel and then passed to the browser.
What are the Disadvantages of ReactJS?
- Lack of proper documentation.
- React libraries evolve constantly which requires learning key aspects again and again.
- JSX can be hard to learn since it mixes JavaScript and ReactJS.
What is Component-Based Architecture?
React speeds up the UI rendering with its unique components-based architecture, this is possible because every component in React is reusable and operates independently of each other, which allows individual small components to render at a faster speed easily.
What are States?
States are a crucial part of React since they are considered source data that controls the component behaviors and their rendering.
Moreover, they create interactive and dynamic UI components.

ReactJS Interview Questions For Senior Developers
If you are a senior developer, then here are some important advanced ReactJS interview questions that you can study.
What is React Hook?
Hooks were introduced in React 16.8. With the help of hooks, you don’t have to write a class and can easily use state and other React features. From function components, they hook into react state and lifecycle features, hence they are known as functions.
Moreover, they are backward compatible, which means they do not come with any breaking changes.
What is Reconciliation?
Reconciliation is a process by which React updates the browser DOM. It is an algorithm that is responsible for which takes decisions on how the render component should be made.
Explain Higher Order Components(HOC).
Higher order component is an advanced technique that allows React to reuse component logic. They can also be used to share the behaviors across all components in React, which increases efficiency and application functioning.
It can be used when:
- Using specific styles for components.
- Rendering components conditionally.
- Bootstrap extraction.
What Are Three Phases Of Component Cycle
Below are the three phases of the component cycle:
Initial Rendering
In the first phase, the UI component begins its journey to the DOM.
Update
In this phase, the component gets updated and rendered again, and then it goes to the DOM.
Unmounting
In this final phase, the component is destroyed and removed from the DOM.
How React is Different from Angular?
Parameter | React | Angular |
---|---|---|
Author | Facebook(Meta) | |
DOM | Virtual DOM | Real DOM |
Architecture | View Layer Of MVC | Complete MVC |
RENDERING | Server Side | Client Side |
Explain Controlled and Uncontrolled Components in React.
Controlled components, also known as dumb components, are capable of changing their state. The data is under the control of the parent component. The ongoing value is fetched with the help of props, which notify changes that occur during callbacks.
Whereas uncontrolled components are the ones who store and manage their states and when you need an ongoing/ current value, you query a DOM using ref and retrieve the value.
Explain Pure Components
These pure components are singular entities that are easy and fast to write. They are capable of replacing components having a render() function, which helps in ensuring the performance of the application is better and the code stays simple.
ReactJS Interview Questions For Experienced
If you are an experienced ReactJS developer, then, our experienced top ReactJS interview questions 2022, which will help you ace any React interview.
Differentiate Between CloneElement and CreateElement
As the name suggests, CloneElement is used to clone an element, and directly pass it to new props.
On the other hand, CreateElement is an entity where JSX is compiled.
What Problems Does React API Context 16 Solve?
Context API allows a way to pass data through the component tree without passing props down at every stage. It’s useful to share data such as user info, UI theme, or any other between child components.
What Problems do React Portal and React Fragment Solve?
Portals are ideal to render children into DOM which is located outside the DOM node hierarchy of the parent component.
Whereas, React Fragment groups children without adding nodes to DOM as React only focuses on returning one object from the render.
What is PropType Library?
PropType is a type checking in react library that exports validators to ensure the data component received is valid. It reduces errors and makes components self-documented.
What Are The Methods of React Component Cycle
componentWillMount():
This function is executed on both the client and server sides before the rendering process.
componentDidMount():
This process is carried out on the client side immediately following the initial render.
componentWillRecieveProps():
When the parent class sends the props and no other render is being called, this function is called.
shouldComponentUpdate():
This Boolean function is responsible for returning true or false as per the scenarios. If the component needs an update, then true is returned, else false is returned.
theComponentWillUpdate():
If the render function is not called, then this function gets called.
componentDidUpdate():
After the render function is called, this function gets called.
componentWillUnmount():
This function is called when a component is unmounted from the DOM.
What is Redux?
Redux is an open JavaScript package that manages the state of an application and creates user interfaces. It allows React components to read from the Redux store, dispatch actions to its store, and update the data.
What is Prop Drilling?
When there is a situation to pass data/state from a top-level component to a deeply nested component, prop drilling is used. It transfers the data/state through intermediate components to an intended destination.
Explain React Fiber
The React fiber is a reimplementation core algorithm and a new engine in React 16. It ensures that React keeps incremental rendering capabilities for virtual DOM. Which helps in increasing the efficiency and performance of UI animations, gestures, etc.
Moreover, it prioritizes updates based on their requirements which further boosts its efficiency.
Enlist Predefined Prop Types
Following are the predefined prop types.
PropTypes.func
- PropTypes.node
- PropTypes.number
- PropTypes.string
- PropTypes.bool
Conclusion
ReactJS is a preferred technology for front-end development. And because it allows mobile and desktop applications to deliver users an intuitive user experience IT companies have a high demand for ReactJS developers.
Though it’s true, IT firms deliberately strategies the hiring process to test candidates’ caliber. And one of the final and major rounds is the technical interview where they will be tested with ReactJS interview questions.
This list of top ReactJS interview questions and answers will give you an idea of what the interviewer will ask and how you can answer to ace the interview.